Note
Go to the end to download the full example code.
Basic Valve Implementation#
This example demonstrates a basic implementation of a valve in Python.
Import necessary libraries#
import os
from PIL import Image
import ansys.mechanical.core as mech
from ansys.mechanical.core.examples import delete_downloads, download_file
from matplotlib import image as mpimg
from matplotlib import pyplot as plt
from matplotlib.animation import FuncAnimation
Embed mechanical and set global variables
app = mech.App(version=241)
globals().update(mech.global_variables(app, True))
print(app)
cwd = os.path.join(os.getcwd(), "out")
def display_image(image_name):
plt.figure(figsize=(16, 9))
plt.imshow(mpimg.imread(os.path.join(cwd, image_name)))
plt.xticks([])
plt.yticks([])
plt.axis("off")
plt.show()
Ansys Mechanical [Ansys Mechanical Enterprise]
Product Version:241
Software build date: 11/27/2023 10:24:20
Configure graphics for image export#
ExtAPI.Graphics.Camera.SetSpecificViewOrientation(ViewOrientationType.Iso)
image_export_format = GraphicsImageExportFormat.PNG
settings_720p = Ansys.Mechanical.Graphics.GraphicsImageExportSettings()
settings_720p.Resolution = GraphicsResolutionType.EnhancedResolution
settings_720p.Background = GraphicsBackgroundType.White
settings_720p.Width = 1280
settings_720p.Height = 720
settings_720p.CurrentGraphicsDisplay = False
Download geometry and import#
Download geometry
geometry_path = download_file("Valve.pmdb", "pymechanical", "embedding")
Import geometry
geometry_import = Model.GeometryImportGroup.AddGeometryImport()
geometry_import_format = (
Ansys.Mechanical.DataModel.Enums.GeometryImportPreference.Format.Automatic
)
geometry_import_preferences = Ansys.ACT.Mechanical.Utilities.GeometryImportPreferences()
geometry_import_preferences.ProcessNamedSelections = True
geometry_import.Import(
geometry_path, geometry_import_format, geometry_import_preferences
)
ExtAPI.Graphics.Camera.SetFit()
ExtAPI.Graphics.ExportImage(
os.path.join(cwd, "geometry.png"), image_export_format, settings_720p
)
display_image("geometry.png")
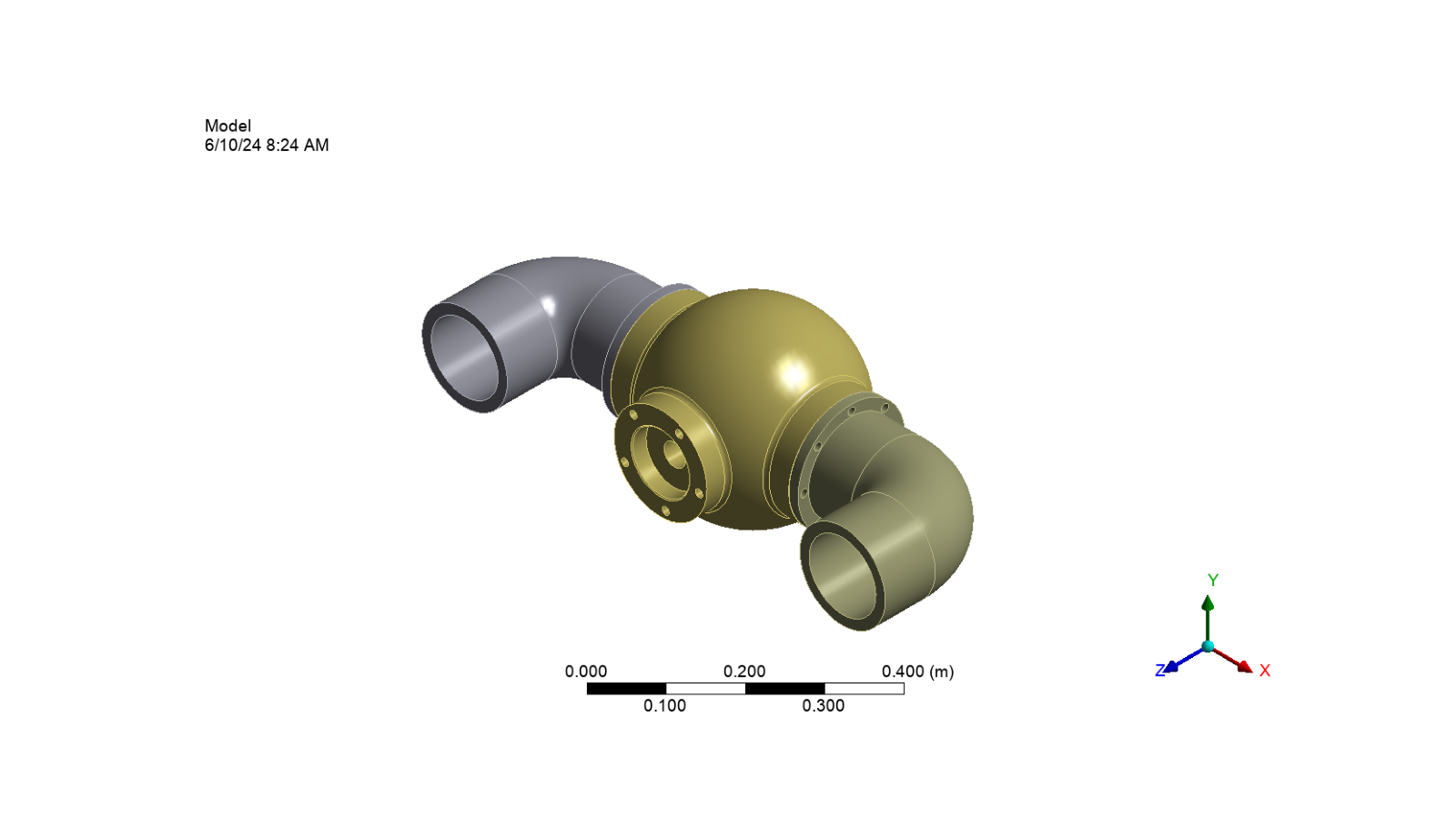
Assign materials and mesh the geometry#
material_assignment = Model.Materials.AddMaterialAssignment()
material_assignment.Material = "Structural Steel"
sel = ExtAPI.SelectionManager.CreateSelectionInfo(
Ansys.ACT.Interfaces.Common.SelectionTypeEnum.GeometryEntities
)
sel.Ids = [
body.GetGeoBody().Id
for body in Model.Geometry.GetChildren(
Ansys.Mechanical.DataModel.Enums.DataModelObjectCategory.Body, True
)
]
material_assignment.Location = sel
Define mesh settings, generate mesh
mesh = Model.Mesh
mesh.ElementSize = Quantity(25, "mm")
mesh.GenerateMesh()
Tree.Activate([mesh])
ExtAPI.Graphics.ExportImage(
os.path.join(cwd, "mesh.png"), image_export_format, settings_720p
)
display_image("mesh.png")
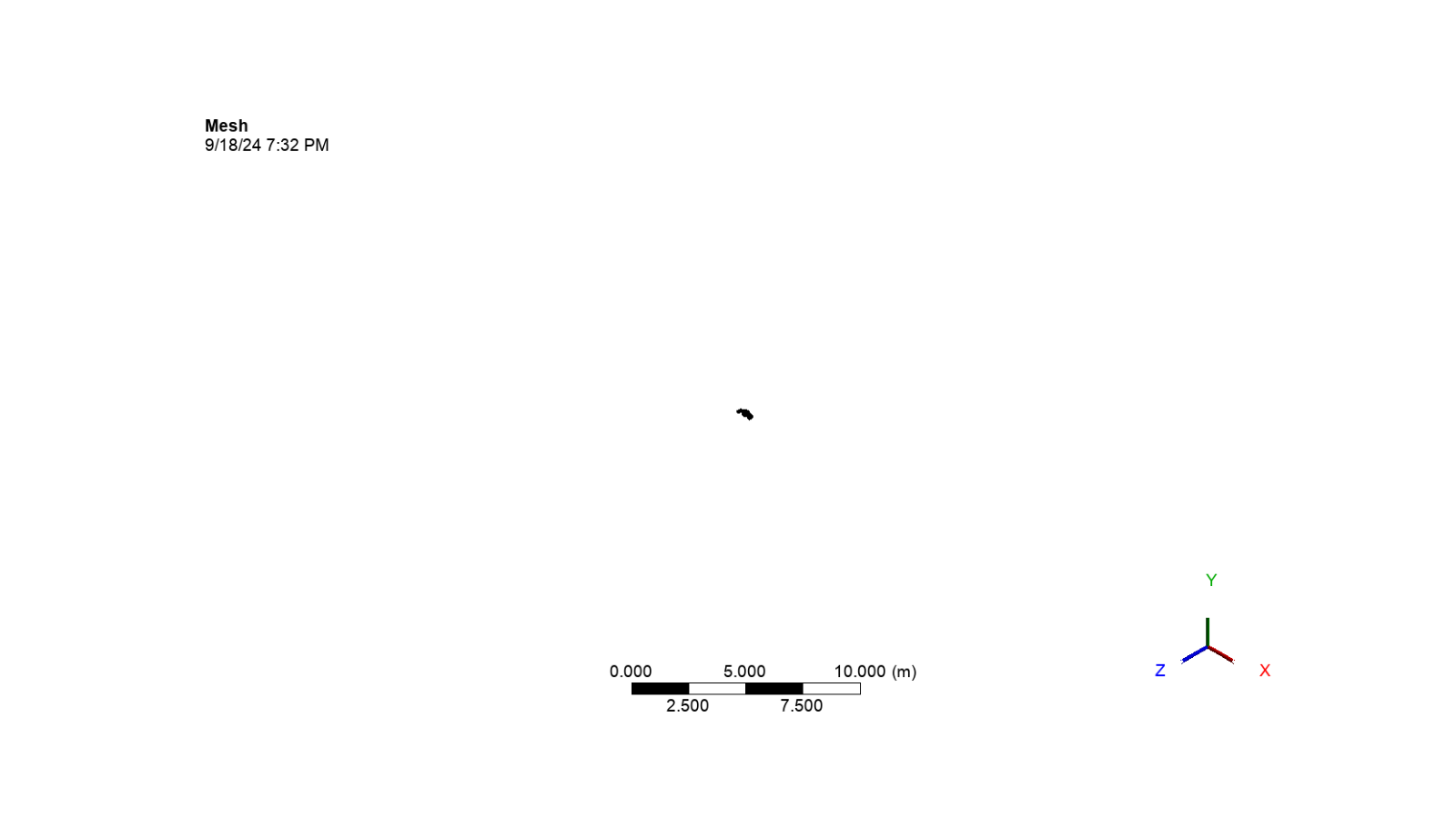
Define analysis and boundary conditions#
analysis = Model.AddStaticStructuralAnalysis()
fixed_support = analysis.AddFixedSupport()
fixed_support.Location = ExtAPI.DataModel.GetObjectsByName("NSFixedSupportFaces")[0]
frictionless_support = analysis.AddFrictionlessSupport()
frictionless_support.Location = ExtAPI.DataModel.GetObjectsByName(
"NSFrictionlessSupportFaces"
)[0]
pressure = analysis.AddPressure()
pressure.Location = ExtAPI.DataModel.GetObjectsByName("NSInsideFaces")[0]
pressure.Magnitude.Inputs[0].DiscreteValues = [Quantity("0 [s]"), Quantity("1 [s]")]
pressure.Magnitude.Output.DiscreteValues = [Quantity("0 [Pa]"), Quantity("15 [MPa]")]
analysis.Activate()
ExtAPI.Graphics.Camera.SetFit()
ExtAPI.Graphics.ExportImage(
os.path.join(cwd, "boundary_conditions.png"), image_export_format, settings_720p
)
display_image("boundary_conditions.png")
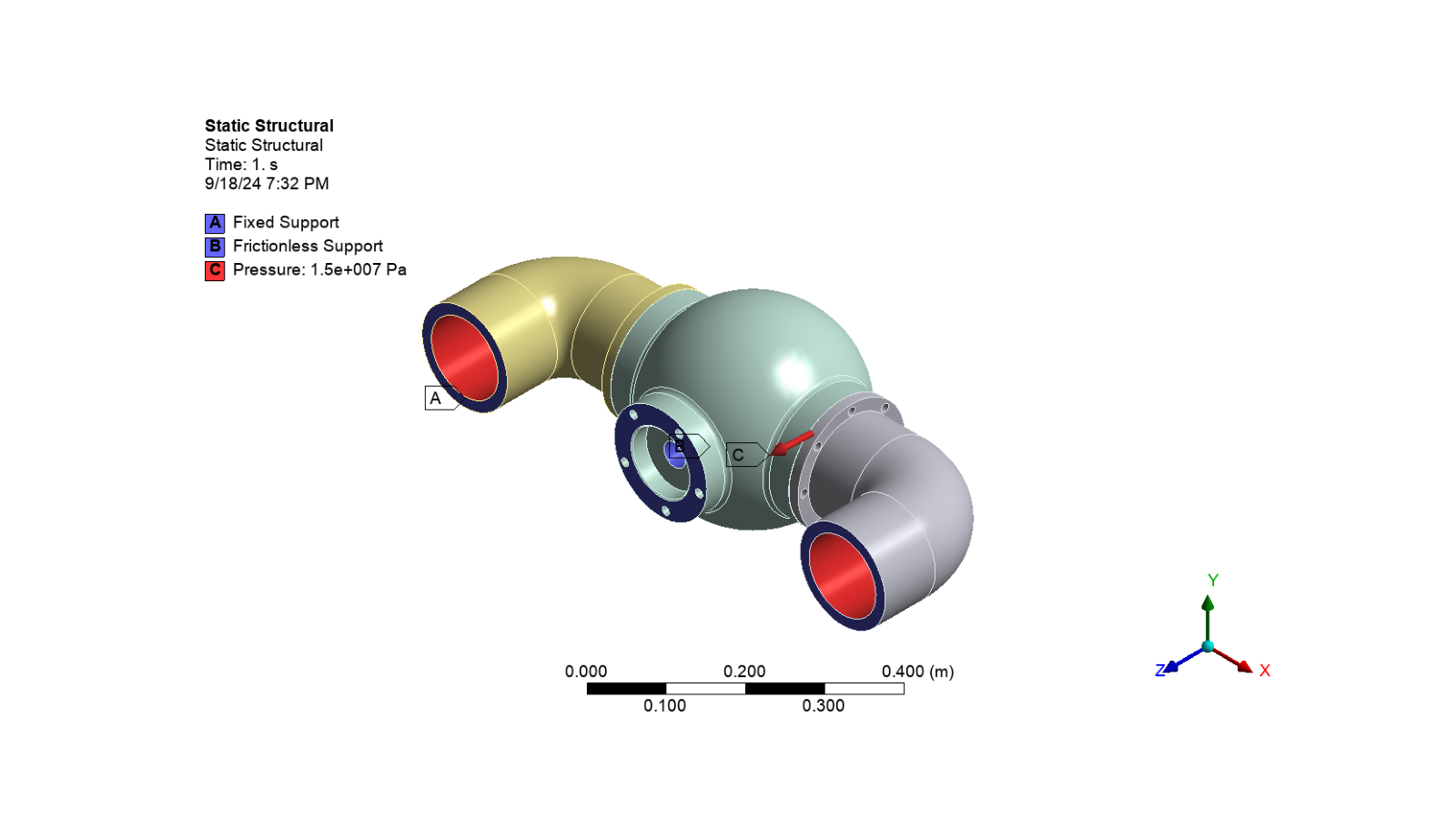
Solve#
Solve process settings
config = ExtAPI.Application.SolveConfigurations["My Computer"]
config.SolveProcessSettings.MaxNumberOfCores = 1
config.SolveProcessSettings.DistributeSolution = False
Add results
solution = analysis.Solution
deformation = solution.AddTotalDeformation()
stress = solution.AddEquivalentStress()
Solve
solution.Solve(True)
Messages#
Messages = ExtAPI.Application.Messages
if Messages:
for message in Messages:
print(f"[{message.Severity}] {message.DisplayString}")
else:
print("No [Info]/[Warning]/[Error] Messages")
No [Info]/[Warning]/[Error] Messages
Results#
def display_image(image_name):
plt.figure(figsize=(16, 9))
plt.imshow(mpimg.imread(os.path.join(cwd, image_name)))
plt.xticks([])
plt.yticks([])
plt.axis("off")
plt.show()
Total deformation
Tree.Activate([deformation])
ExtAPI.Graphics.ExportImage(
os.path.join(cwd, "totaldeformation_valve.png"), image_export_format, settings_720p
)
display_image("totaldeformation_valve.png")
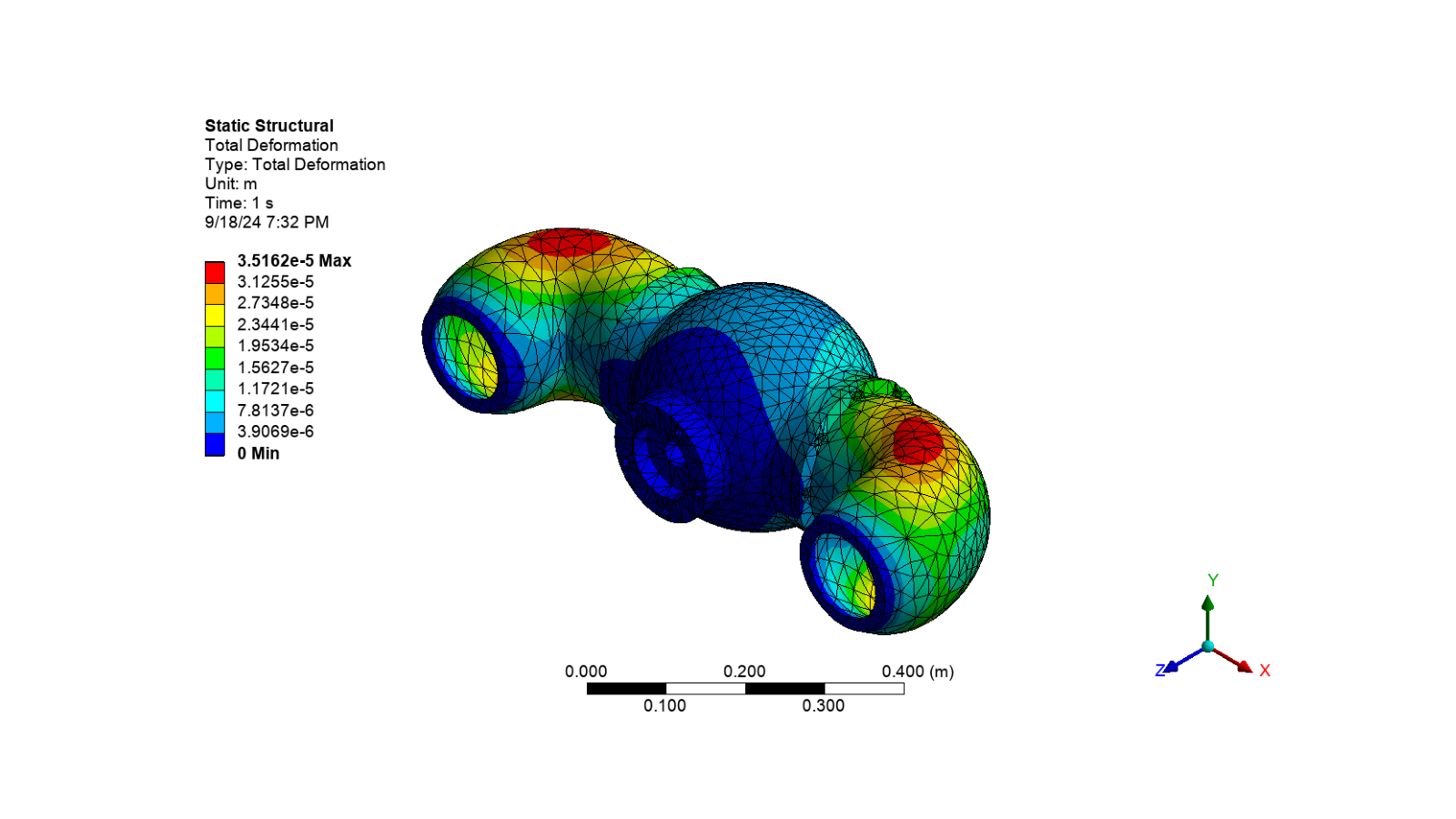
Stress
Tree.Activate([stress])
ExtAPI.Graphics.ExportImage(
os.path.join(cwd, "stress_valve.png"), image_export_format, settings_720p
)
display_image("stress_valve.png")
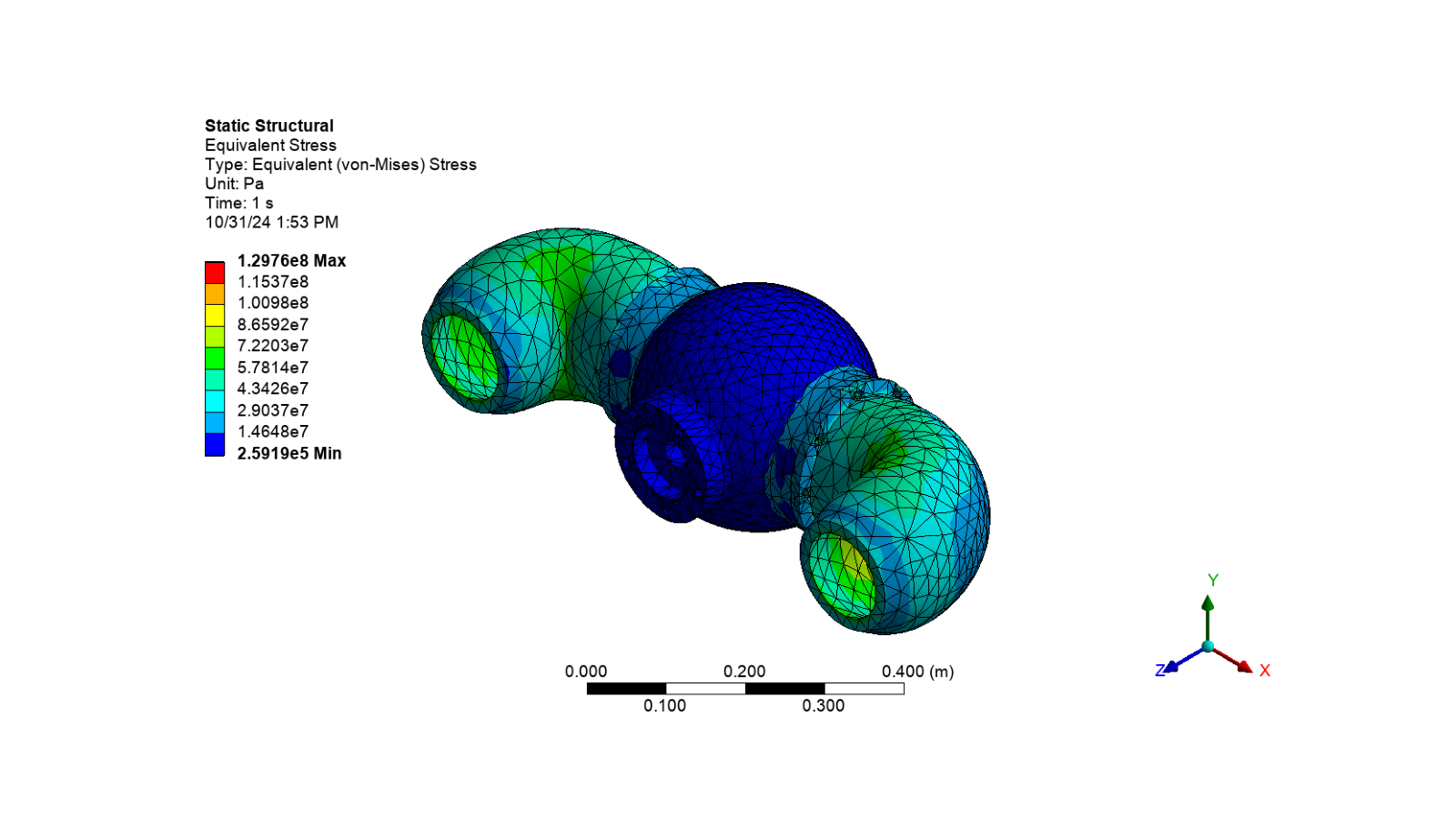
Export stress animation
animation_export_format = (
Ansys.Mechanical.DataModel.Enums.GraphicsAnimationExportFormat.GIF
)
settings_720p = Ansys.Mechanical.Graphics.AnimationExportSettings()
settings_720p.Width = 1280
settings_720p.Height = 720
stress.ExportAnimation(
os.path.join(cwd, "Valve.gif"), animation_export_format, settings_720p
)
gif = Image.open(os.path.join(cwd, "Valve.gif"))
fig, ax = plt.subplots(figsize=(16, 9))
ax.axis("off")
img = ax.imshow(gif.convert("RGBA"))
def update(frame):
gif.seek(frame)
img.set_array(gif.convert("RGBA"))
return [img]
ani = FuncAnimation(
fig, update, frames=range(gif.n_frames), interval=100, repeat=True, blit=True
)
plt.show()
Display output file from solve#
def write_file_contents_to_console(path):
"""Write file contents to console."""
with open(path, "rt") as file:
for line in file:
print(line, end="")
solve_path = analysis.WorkingDir
solve_out_path = os.path.join(solve_path, "solve.out")
if solve_out_path:
write_file_contents_to_console(solve_out_path)
Ansys Mechanical Enterprise
*------------------------------------------------------------------*
| |
| W E L C O M E T O T H E A N S Y S (R) P R O G R A M |
| |
*------------------------------------------------------------------*
***************************************************************
* ANSYS MAPDL 2024 R1 LEGAL NOTICES *
***************************************************************
* *
* Copyright 1971-2024 Ansys, Inc. All rights reserved. *
* Unauthorized use, distribution or duplication is *
* prohibited. *
* *
* Ansys is a registered trademark of Ansys, Inc. or its *
* subsidiaries in the United States or other countries. *
* See the Ansys, Inc. online documentation or the Ansys, Inc. *
* documentation CD or online help for the complete Legal *
* Notice. *
* *
***************************************************************
* *
* THIS ANSYS SOFTWARE PRODUCT AND PROGRAM DOCUMENTATION *
* INCLUDE TRADE SECRETS AND CONFIDENTIAL AND PROPRIETARY *
* PRODUCTS OF ANSYS, INC., ITS SUBSIDIARIES, OR LICENSORS. *
* The software products and documentation are furnished by *
* Ansys, Inc. or its subsidiaries under a software license *
* agreement that contains provisions concerning *
* non-disclosure, copying, length and nature of use, *
* compliance with exporting laws, warranties, disclaimers, *
* limitations of liability, and remedies, and other *
* provisions. The software products and documentation may be *
* used, disclosed, transferred, or copied only in accordance *
* with the terms and conditions of that software license *
* agreement. *
* *
* Ansys, Inc. is a UL registered *
* ISO 9001:2015 company. *
* *
***************************************************************
* *
* This product is subject to U.S. laws governing export and *
* re-export. *
* *
* For U.S. Government users, except as specifically granted *
* by the Ansys, Inc. software license agreement, the use, *
* duplication, or disclosure by the United States Government *
* is subject to restrictions stated in the Ansys, Inc. *
* software license agreement and FAR 12.212 (for non-DOD *
* licenses). *
* *
***************************************************************
AFTER YOU HAVE READ, UNDERSTOOD, AND AGREED TO THE PREVIOUS NOTICES,
PRESS <CR> OR <ENTER> TO CONTINUE
2024 R1
Point Releases and Patches installed:
Ansys, Inc. License Manager 2024 R1
Structures 2024 R1
LS-DYNA 2024 R1
Mechanical Products 2024 R1
***** MAPDL COMMAND LINE ARGUMENTS *****
BATCH MODE REQUESTED (-b) = NOLIST
INPUT FILE COPY MODE (-c) = COPY
SHARED MEMORY PARALLEL REQUESTED
SINGLE PROCESS WITH SINGLE THREAD REQUESTED
TOTAL OF 1 CORES REQUESTED
INPUT FILE NAME = /github/home/.mw/Application Data/Ansys/v241/AnsysMech4328/Project_Mech_Files/StaticStructural/dummy.dat
OUTPUT FILE NAME = /github/home/.mw/Application Data/Ansys/v241/AnsysMech4328/Project_Mech_Files/StaticStructural/solve.out
START-UP FILE MODE = NOREAD
STOP FILE MODE = NOREAD
RELEASE= 2024 R1 BUILD= 24.1 UP20231106 VERSION=LINUX x64
CURRENT JOBNAME=file 07:43:16 MAY 06, 2024 CP= 0.223
PARAMETER _DS_PROGRESS = 999.0000000
/INPUT FILE= ds.dat LINE= 0
DO NOT WRITE ELEMENT RESULTS INTO DATABASE
*GET _WALLSTRT FROM ACTI ITEM=TIME WALL VALUE= 7.72111111
TITLE=
--Static Structural
SET PARAMETER DIMENSIONS ON _WB_PROJECTSCRATCH_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_PROJECTSCRATCH_DIR(1) = /github/home/.mw/Application Data/Ansys/v241/AnsysMech4328/Project_Mech_Files/StaticStructural/
SET PARAMETER DIMENSIONS ON _WB_SOLVERFILES_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_SOLVERFILES_DIR(1) = /github/home/.mw/Application Data/Ansys/v241/AnsysMech4328/Project_Mech_Files/StaticStructural/
SET PARAMETER DIMENSIONS ON _WB_USERFILES_DIR
TYPE=STRI DIMENSIONS= 248 1 1
PARAMETER _WB_USERFILES_DIR(1) = /github/home/.mw/Application Data/Ansys/v241/AnsysMech4328/Project_Mech_Files/UserFiles/
--- Data in consistent MKS units. See Solving Units in the help system for more
MKS UNITS SPECIFIED FOR INTERNAL
LENGTH (l) = METER (M)
MASS (M) = KILOGRAM (KG)
TIME (t) = SECOND (SEC)
TEMPERATURE (T) = CELSIUS (C)
TOFFSET = 273.0
CHARGE (Q) = COULOMB
FORCE (f) = NEWTON (N) (KG-M/SEC2)
HEAT = JOULE (N-M)
PRESSURE = PASCAL (NEWTON/M**2)
ENERGY (W) = JOULE (N-M)
POWER (P) = WATT (N-M/SEC)
CURRENT (i) = AMPERE (COULOMBS/SEC)
CAPACITANCE (C) = FARAD
INDUCTANCE (L) = HENRY
MAGNETIC FLUX = WEBER
RESISTANCE (R) = OHM
ELECTRIC POTENTIAL = VOLT
INPUT UNITS ARE ALSO SET TO MKS
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R1 24.1 ***
Ansys Mechanical Enterprise
00000000 VERSION=LINUX x64 07:43:16 MAY 06, 2024 CP= 0.247
--Static Structural
***** MAPDL ANALYSIS DEFINITION (PREP7) *****
*********** Nodes for the whole assembly ***********
*********** Elements for Body 1 "Connector\Solid1" ***********
*********** Elements for Body 2 "Right_elbow\Solid1" ***********
*********** Elements for Body 3 "Left_elbow\Solid1" ***********
*********** Send User Defined Coordinate System(s) ***********
*********** Set Reference Temperature ***********
*********** Send Materials ***********
*********** Create Contact "Contact Region" ***********
Real Constant Set For Above Contact Is 5 & 4
*********** Create Contact "Contact Region 2" ***********
Real Constant Set For Above Contact Is 7 & 6
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Send Named Selection as Node Component ***********
*********** Fixed Supports ***********
********* Frictionless Supports X *********
********* Frictionless Supports Z *********
*********** Node Rotations ***********
*********** Define Pressure Using Surface Effect Elements "Pressure" **********
***** ROUTINE COMPLETED ***** CP = 0.359
--- Number of total nodes = 26882
--- Number of contact elements = 3294
--- Number of spring elements = 0
--- Number of bearing elements = 0
--- Number of solid elements = 14427
--- Number of condensed parts = 0
--- Number of total elements = 17721
*GET _WALLBSOL FROM ACTI ITEM=TIME WALL VALUE= 7.72111111
****************************************************************************
************************* SOLUTION ********************************
****************************************************************************
***** MAPDL SOLUTION ROUTINE *****
PERFORM A STATIC ANALYSIS
THIS WILL BE A NEW ANALYSIS
PARAMETER _THICKRATIO = 0.3330000000
USE SPARSE MATRIX DIRECT SOLVER
CONTACT INFORMATION PRINTOUT LEVEL 1
NLDIAG: Nonlinear diagnostics CONT option is set to ON.
Writing frequency : each ITERATION.
DO NOT SAVE ANY RESTART FILES AT ALL
****************************************************
******************* SOLVE FOR LS 1 OF 1 ****************
SELECT FOR ITEM=TYPE COMPONENT=
IN RANGE 8 TO 8 STEP 1
1694 ELEMENTS (OF 17721 DEFINED) SELECTED BY ESEL COMMAND.
SELECT ALL NODES HAVING ANY ELEMENT IN ELEMENT SET.
3556 NODES (OF 26882 DEFINED) SELECTED FROM
1694 SELECTED ELEMENTS BY NSLE COMMAND.
GENERATE SURFACE LOAD PRES ON SURFACE DEFINED BY ALL SELECTED NODES
SET ACCORDING TO TABLE PARAMETER = _LOADVARI56
NUMBER OF PRES ELEMENT FACE LOADS STORED = 1694
ALL SELECT FOR ITEM=NODE COMPONENT=
IN RANGE 1 TO 26882 STEP 1
26882 NODES (OF 26882 DEFINED) SELECTED BY NSEL COMMAND.
ALL SELECT FOR ITEM=ELEM COMPONENT=
IN RANGE 1 TO 25061 STEP 1
17721 ELEMENTS (OF 17721 DEFINED) SELECTED BY ESEL COMMAND.
ALL SELECT FOR ITEM=ELEM COMPONENT=
IN RANGE 1 TO 25061 STEP 1
17721 ELEMENTS (OF 17721 DEFINED) SELECTED BY ESEL COMMAND.
PRINTOUT RESUMED BY /GOP
USE 1 SUBSTEPS INITIALLY THIS LOAD STEP FOR ALL DEGREES OF FREEDOM
FOR AUTOMATIC TIME STEPPING:
USE 1 SUBSTEPS AS A MAXIMUM
USE 1 SUBSTEPS AS A MINIMUM
TIME= 1.0000
ERASE THE CURRENT DATABASE OUTPUT CONTROL TABLE.
WRITE ALL ITEMS TO THE DATABASE WITH A FREQUENCY OF NONE
FOR ALL APPLICABLE ENTITIES
WRITE NSOL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE RSOL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EANG ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE ETMP ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE VENG ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE STRS ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EPEL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE EPPL ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
WRITE CONT ITEMS TO THE DATABASE WITH A FREQUENCY OF ALL
FOR ALL APPLICABLE ENTITIES
*GET ANSINTER_ FROM ACTI ITEM=INT VALUE= 0.00000000
*IF ANSINTER_ ( = 0.00000 ) NE
0 ( = 0.00000 ) THEN
*ENDIF
***** MAPDL SOLVE COMMAND *****
*** WARNING *** CP = 0.397 TIME= 07:43:16
Element shape checking is currently inactive. Issue SHPP,ON or
SHPP,WARN to reactivate, if desired.
*** NOTE *** CP = 0.415 TIME= 07:43:16
The model data was checked and warning messages were found.
Please review output or errors file ( /github/home/.mw/Application
Data/Ansys/v241/AnsysMech4328/Project_Mech_Files/StaticStructural/file.
.err ) for these warning messages.
*** SELECTION OF ELEMENT TECHNOLOGIES FOR APPLICABLE ELEMENTS ***
--- GIVE SUGGESTIONS AND RESET THE KEY OPTIONS ---
ELEMENT TYPE 1 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 2 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
ELEMENT TYPE 3 IS SOLID187. IT IS NOT ASSOCIATED WITH FULLY INCOMPRESSIBLE
HYPERELASTIC MATERIALS. NO SUGGESTION IS AVAILABLE AND NO RESETTING IS NEEDED.
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R1 24.1 ***
Ansys Mechanical Enterprise
00000000 VERSION=LINUX x64 07:43:16 MAY 06, 2024 CP= 0.418
--Static Structural
S O L U T I O N O P T I O N S
PROBLEM DIMENSIONALITY. . . . . . . . . . . . .3-D
DEGREES OF FREEDOM. . . . . . UX UY UZ
ANALYSIS TYPE . . . . . . . . . . . . . . . . .STATIC (STEADY-STATE)
OFFSET TEMPERATURE FROM ABSOLUTE ZERO . . . . . 273.15
EQUATION SOLVER OPTION. . . . . . . . . . . . .SPARSE
GLOBALLY ASSEMBLED MATRIX . . . . . . . . . . .SYMMETRIC
*** WARNING *** CP = 0.427 TIME= 07:43:16
Material number 8 (used by element 23368) should normally have at least
one MP or one TB type command associated with it. Output of energy by
material may not be available.
*** NOTE *** CP = 0.436 TIME= 07:43:16
The step data was checked and warning messages were found.
Please review output or errors file ( /github/home/.mw/Application
Data/Ansys/v241/AnsysMech4328/Project_Mech_Files/StaticStructural/file.
.err ) for these warning messages.
*** NOTE *** CP = 0.436 TIME= 07:43:16
The conditions for direct assembly have been met. No .emat or .erot
files will be produced.
L O A D S T E P O P T I O N S
LOAD STEP NUMBER. . . . . . . . . . . . . . . . 1
TIME AT END OF THE LOAD STEP. . . . . . . . . . 1.0000
NUMBER OF SUBSTEPS. . . . . . . . . . . . . . . 1
STEP CHANGE BOUNDARY CONDITIONS . . . . . . . . NO
PRINT OUTPUT CONTROLS . . . . . . . . . . . . .NO PRINTOUT
DATABASE OUTPUT CONTROLS
ITEM FREQUENCY COMPONENT
ALL NONE
NSOL ALL
RSOL ALL
EANG ALL
ETMP ALL
VENG ALL
STRS ALL
EPEL ALL
EPPL ALL
CONT ALL
SOLUTION MONITORING INFO IS WRITTEN TO FILE= file.mntr
*** NOTE *** CP = 0.719 TIME= 07:43:16
Symmetric Deformable- deformable contact pair identified by real
constant set 4 and contact element type 4 has been set up. The
companion pair has real constant set ID 5. Both pairs should have the
same behavior.
MAPDL will deactivate the current pair and keep its companion pair,
resulting in asymmetric contact.
Auto surface constraint is built
Contact algorithm: MPC based approach
*** NOTE *** CP = 0.719 TIME= 07:43:16
Contact related postprocess items (ETABLE, pressure ...) are not
available.
Contact detection at: nodal point (normal to target surface)
MPC will be built internally to handle bonded contact.
Average contact surface length 0.14109E-01
Average contact pair depth 0.93576E-02
Average target surface length 0.13700E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.23394E-02
Default target edge extension factor TOLS 2.0000
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 0.720 TIME= 07:43:16
Max. Initial penetration 7.571439156E-17 was detected between contact
element 21972 and target element 22406.
****************************************
*** NOTE *** CP = 0.720 TIME= 07:43:16
Symmetric Deformable- deformable contact pair identified by real
constant set 5 and contact element type 4 has been set up. The
companion pair has real constant set ID 4. Both pairs should have the
same behavior.
MAPDL will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Auto surface constraint is built
Contact algorithm: MPC based approach
*** NOTE *** CP = 0.720 TIME= 07:43:16
Contact related postprocess items (ETABLE, pressure ...) are not
available.
Contact detection at: nodal point (normal to target surface)
MPC will be built internally to handle bonded contact.
Average contact surface length 0.14033E-01
Average contact pair depth 0.82697E-02
Average target surface length 0.13762E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.20674E-02
Default target edge extension factor TOLS 2.0000
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 0.720 TIME= 07:43:16
Max. Initial penetration 8.326672685E-17 was detected between contact
element 22262 and target element 21953.
****************************************
*** NOTE *** CP = 0.720 TIME= 07:43:16
Symmetric Deformable- deformable contact pair identified by real
constant set 6 and contact element type 6 has been set up. The
companion pair has real constant set ID 7. Both pairs should have the
same behavior.
*WARNING*: The contact pairs have similar mesh patterns which can cause
overconstraint. MAPDL will deactivate the current pair and keep its
companion pair.
Contact algorithm: MPC based approach
*** NOTE *** CP = 0.720 TIME= 07:43:16
Contact related postprocess items (ETABLE, pressure ...) are not
available.
Contact detection at: nodal point (normal to target surface)
MPC will be built internally to handle bonded contact.
Average contact surface length 0.14109E-01
Average contact pair depth 0.91767E-02
Average target surface length 0.13770E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.22942E-02
Default target edge extension factor TOLS 2.0000
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 0.720 TIME= 07:43:16
Max. Initial penetration 8.326672685E-17 was detected between contact
element 22936 and target element 23341.
****************************************
*** NOTE *** CP = 0.720 TIME= 07:43:16
Symmetric Deformable- deformable contact pair identified by real
constant set 7 and contact element type 6 has been set up. The
companion pair has real constant set ID 6. Both pairs should have the
same behavior.
MAPDL will keep the current pair and deactivate its companion pair,
resulting in asymmetric contact.
Auto surface constraint is built
Contact algorithm: MPC based approach
*** NOTE *** CP = 0.720 TIME= 07:43:16
Contact related postprocess items (ETABLE, pressure ...) are not
available.
Contact detection at: nodal point (normal to target surface)
MPC will be built internally to handle bonded contact.
Average contact surface length 0.14121E-01
Average contact pair depth 0.81425E-02
Average target surface length 0.13762E-01
Default pinball region factor PINB 0.25000
The resulting pinball region 0.20356E-02
Default target edge extension factor TOLS 2.0000
Initial penetration/gap is excluded.
Bonded contact (always) is defined.
*** NOTE *** CP = 0.720 TIME= 07:43:16
Max. Initial penetration 8.326672685E-17 was detected between contact
element 22977 and target element 22681.
****************************************
The FEA model contains 0 external CE equations and 2829 internal CE
equations.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 4
*** NOTE *** CP = 1.747 TIME= 07:43:17
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 5
Max. Penetration of 0 has been detected between contact element 22168
and target element 21830.
Max. Geometrical gap of 8.326672685E-17 has been detected between
contact element 22235 and target element 21774.
Max. Geometrical penetration of -8.326672685E-17 has been detected
between contact element 22235 and target element 21774.
For total 200 contact elements, there are 200 elements are in contact.
There are 200 elements are in sticking.
Max. Pinball distance 2.067419789E-03.
One of the contact searching regions contains at least 20 target
elements.
*************************************************
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 6
*** NOTE *** CP = 1.747 TIME= 07:43:17
Contact pair is inactive.
*************************************************
SUMMARY FOR CONTACT PAIR IDENTIFIED BY REAL CONSTANT SET 7
Max. Penetration of 0 has been detected between contact element 22968
and target element 22572.
Max. Geometrical gap of 8.326672685E-17 has been detected between
contact element 23082 and target element 22663.
Max. Geometrical penetration of -7.806255642E-17 has been detected
between contact element 23082 and target element 22663.
For total 200 contact elements, there are 200 elements are in contact.
There are 200 elements are in sticking.
Max. Pinball distance 2.035619409E-03.
One of the contact searching regions contains at least 20 target
elements.
*************************************************
*********** PRECISE MASS SUMMARY ***********
TOTAL RIGID BODY MASS MATRIX ABOUT ORIGIN
Translational mass | Coupled translational/rotational mass
138.29 0.0000 0.0000 | 0.0000 -56.537 30.296
0.0000 138.29 0.0000 | 56.537 0.0000 0.73727E-02
0.0000 0.0000 138.29 | -30.296 -0.73727E-02 0.0000
------------------------------------------ | ------------------------------------------
| Rotational mass (inertia)
| 31.211 0.16326E-02 0.30943E-02
| 0.16326E-02 27.754 -12.386
| 0.30943E-02 -12.386 11.103
TOTAL MASS = 138.29
The mass principal axes coincide with the global Cartesian axes
CENTER OF MASS (X,Y,Z)= 0.53313E-04 -0.21907 -0.40882
TOTAL INERTIA ABOUT CENTER OF MASS
1.4604 0.17394E-04 0.80195E-04
0.17394E-04 4.6403 0.15516E-05
0.80195E-04 0.15516E-05 4.4656
The inertia principal axes coincide with the global Cartesian axes
*** MASS SUMMARY BY ELEMENT TYPE ***
TYPE MASS
1 100.182
2 19.0548
3 19.0556
Range of element maximum matrix coefficients in global coordinates
Maximum = 2.93408494E+10 at element 11441.
Minimum = 518803319 at element 2355.
*** ELEMENT MATRIX FORMULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 8724 SOLID187 0.396 0.000045
2 2759 SOLID187 0.127 0.000046
3 2944 SOLID187 0.134 0.000045
4 400 CONTA174 0.051 0.000127
5 400 TARGE170 0.001 0.000003
6 400 CONTA174 0.050 0.000126
7 400 TARGE170 0.001 0.000002
8 1694 SURF154 0.051 0.000030
Time at end of element matrix formulation CP = 1.74807906.
SPARSE MATRIX DIRECT SOLVER.
Number of equations = 76728, Maximum wavefront = 480
Memory allocated for solver = 397.284 MB
Memory required for in-core solution = 380.954 MB
Memory required for out-of-core solution = 148.156 MB
*** NOTE *** CP = 2.238 TIME= 07:43:18
The Sparse Matrix Solver is currently running in the in-core memory
mode. This memory mode uses the most amount of memory in order to
avoid using the hard drive as much as possible, which most often
results in the fastest solution time. This mode is recommended if
enough physical memory is present to accommodate all of the solver
data.
curEqn= 38063 totEqn= 76728 Job CP sec= 2.603
Factor Done= 56% Factor Wall sec= 0.423 rate= 30.7 GFlops
curEqn= 76728 totEqn= 76728 Job CP sec= 2.954
Factor Done= 100% Factor Wall sec= 0.772 rate= 30.1 GFlops
Sparse solver maximum pivot= 3.152432104E+10 at node 19308 UZ.
Sparse solver minimum pivot= 245329060 at node 15632 UY.
Sparse solver minimum pivot in absolute value= 245329060 at node 15632
UY.
*** ELEMENT RESULT CALCULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 8724 SOLID187 0.357 0.000041
2 2759 SOLID187 0.114 0.000041
3 2944 SOLID187 0.121 0.000041
4 400 CONTA174 0.007 0.000017
6 400 CONTA174 0.007 0.000017
8 1694 SURF154 0.040 0.000024
*** NODAL LOAD CALCULATION TIMES
TYPE NUMBER ENAME TOTAL CP AVE CP
1 8724 SOLID187 0.118 0.000013
2 2759 SOLID187 0.038 0.000014
3 2944 SOLID187 0.040 0.000013
4 400 CONTA174 0.001 0.000003
6 400 CONTA174 0.001 0.000003
8 1694 SURF154 0.006 0.000003
*** LOAD STEP 1 SUBSTEP 1 COMPLETED. CUM ITER = 1
*** TIME = 1.00000 TIME INC = 1.00000 NEW TRIANG MATRIX
*** MAPDL BINARY FILE STATISTICS
BUFFER SIZE USED= 16384
18.062 MB WRITTEN ON ELEMENT SAVED DATA FILE: file.esav
40.625 MB WRITTEN ON ASSEMBLED MATRIX FILE: file.full
9.875 MB WRITTEN ON RESULTS FILE: file.rst
*************** Write FE CONNECTORS *********
WRITE OUT CONSTRAINT EQUATIONS TO FILE= file.ce
****************************************************
*************** FINISHED SOLVE FOR LS 1 *************
*GET _WALLASOL FROM ACTI ITEM=TIME WALL VALUE= 7.72222222
PRINTOUT RESUMED BY /GOP
FINISH SOLUTION PROCESSING
***** ROUTINE COMPLETED ***** CP = 4.378
*** MAPDL - ENGINEERING ANALYSIS SYSTEM RELEASE 2024 R1 24.1 ***
Ansys Mechanical Enterprise
00000000 VERSION=LINUX x64 07:43:20 MAY 06, 2024 CP= 4.379
--Static Structural
***** MAPDL RESULTS INTERPRETATION (POST1) *****
*** NOTE *** CP = 4.379 TIME= 07:43:20
Reading results into the database (SET command) will update the current
displacement and force boundary conditions in the database with the
values from the results file for that load set. Note that any
subsequent solutions will use these values unless action is taken to
either SAVE the current values or not overwrite them (/EXIT,NOSAVE).
Set Encoding of XML File to:ISO-8859-1
Set Output of XML File to:
PARM, , , , , , , , , , , ,
, , , , , , ,
DATABASE WRITTEN ON FILE parm.xml
EXIT THE MAPDL POST1 DATABASE PROCESSOR
***** ROUTINE COMPLETED ***** CP = 4.379
PRINTOUT RESUMED BY /GOP
*GET _WALLDONE FROM ACTI ITEM=TIME WALL VALUE= 7.72222222
PARAMETER _PREPTIME = 0.000000000
PARAMETER _SOLVTIME = 4.000000000
PARAMETER _POSTTIME = 0.000000000
PARAMETER _TOTALTIM = 4.000000000
*GET _DLBRATIO FROM ACTI ITEM=SOLU DLBR VALUE= 0.00000000
*GET _COMBTIME FROM ACTI ITEM=SOLU COMB VALUE= 0.00000000
*GET _SSMODE FROM ACTI ITEM=SOLU SSMM VALUE= 2.00000000
*GET _NDOFS FROM ACTI ITEM=SOLU NDOF VALUE= 76728.0000
--- Total number of nodes = 26882
--- Total number of elements = 17721
--- Element load balance ratio = 0
--- Time to combine distributed files = 0
--- Sparse memory mode = 2
--- Number of DOF = 76728
EXIT MAPDL WITHOUT SAVING DATABASE
NUMBER OF WARNING MESSAGES ENCOUNTERED= 2
NUMBER OF ERROR MESSAGES ENCOUNTERED= 0
+--------------------- M A P D L S T A T I S T I C S ------------------------+
Release: 2024 R1 Build: 24.1 Update: UP20231106 Platform: LINUX x64
Date Run: 05/06/2024 Time: 07:43 Process ID: 13129
Operating System: Ubuntu 20.04.6 LTS
Processor Model: AMD EPYC 7763 64-Core Processor
Compiler: Intel(R) Fortran Compiler Classic Version 2021.9 (Build: 20230302)
Intel(R) C/C++ Compiler Classic Version 2021.9 (Build: 20230302)
Intel(R) Math Kernel Library Version 2020.0.0 Product Build 20191122
BLAS Library supplied by AMD BLIS
Number of machines requested : 1
Total number of cores available : 8
Number of physical cores available : 4
Number of processes requested : 1
Number of threads per process requested : 1
Total number of cores requested : 1 (Shared Memory Parallel)
GPU Acceleration: Not Requested
Job Name: file
Input File: dummy.dat
Working Directory: /github/home/.mw/Application Data/Ansys/v241/AnsysMech4328/Project_Mech_Files/StaticStructural
Total CPU time for main thread : 4.4 seconds
Total CPU time summed for all threads : 4.4 seconds
Elapsed time spent obtaining a license : 0.2 seconds
Elapsed time spent pre-processing model (/PREP7) : 0.1 seconds
Elapsed time spent solution - preprocessing : 0.2 seconds
Elapsed time spent computing solution : 3.7 seconds
Elapsed time spent solution - postprocessing : 0.0 seconds
Elapsed time spent post-processing model (/POST1) : 0.0 seconds
Equation solver used : Sparse (symmetric)
Equation solver computational rate : 30.1 Gflops
Sum of disk space used on all processes : 73.7 MB
Sum of memory used on all processes : 512.0 MB
Sum of memory allocated on all processes : 2112.0 MB
Physical memory available : 31 GB
Total amount of I/O written to disk : 0.1 GB
Total amount of I/O read from disk : 0.0 GB
+------------------ E N D M A P D L S T A T I S T I C S -------------------+
*-----------------------------------------------------------------------------*
| |
| RUN COMPLETED |
| |
|-----------------------------------------------------------------------------|
| |
| Ansys MAPDL 2024 R1 Build 24.1 UP20231106 LINUX x64 |
| |
|-----------------------------------------------------------------------------|
| |
| Database Requested(-db) 1024 MB Scratch Memory Requested 1024 MB |
| Maximum Database Used 23 MB Maximum Scratch Memory Used 489 MB |
| |
|-----------------------------------------------------------------------------|
| |
| CP Time (sec) = 4.388 Time = 07:43:20 |
| Elapsed Time (sec) = 5.000 Date = 05/06/2024 |
| |
*-----------------------------------------------------------------------------*
Project tree#
def print_tree(node, indentation=""):
print(f"{indentation}├── {node.Name}")
if (
hasattr(node, "Children")
and node.Children is not None
and node.Children.Count > 0
):
for child in node.Children:
print_tree(child, indentation + "| ")
root_node = DataModel.Project
print_tree(root_node)
├── Project
| ├── Model
| | ├── Geometry Imports
| | | ├── Geometry Import
| | ├── Geometry
| | | ├── Connector
| | | | ├── Connector\Solid1
| | | ├── Right_elbow
| | | | ├── Right_elbow\Solid1
| | | ├── Left_elbow
| | | | ├── Left_elbow\Solid1
| | ├── Materials
| | | ├── Structural Steel
| | | ├── Structural Steel Assignment
| | ├── Coordinate Systems
| | | ├── Global Coordinate System
| | ├── Remote Points
| | ├── Connections
| | | ├── Contacts
| | | | ├── Contact Region
| | | | ├── Contact Region 2
| | ├── Mesh
| | ├── Named Selections
| | | ├── NSFixedSupportFaces
| | | ├── NSFrictionlessSupportFaces
| | | ├── NSInsideFaces
| | ├── Static Structural
| | | ├── Analysis Settings
| | | ├── Fixed Support
| | | ├── Frictionless Support
| | | ├── Pressure
| | | ├── Solution
| | | | ├── Solution Information
| | | | ├── Total Deformation
| | | | ├── Equivalent Stress
Cleanup#
Save project
app.save(os.path.join(cwd, "Valve.mechdat"))
app.new()
delete example files
delete_downloads()
True
Total running time of the script: (0 minutes 25.394 seconds)